How to use and set up Tailwind CSS with Nextjs.
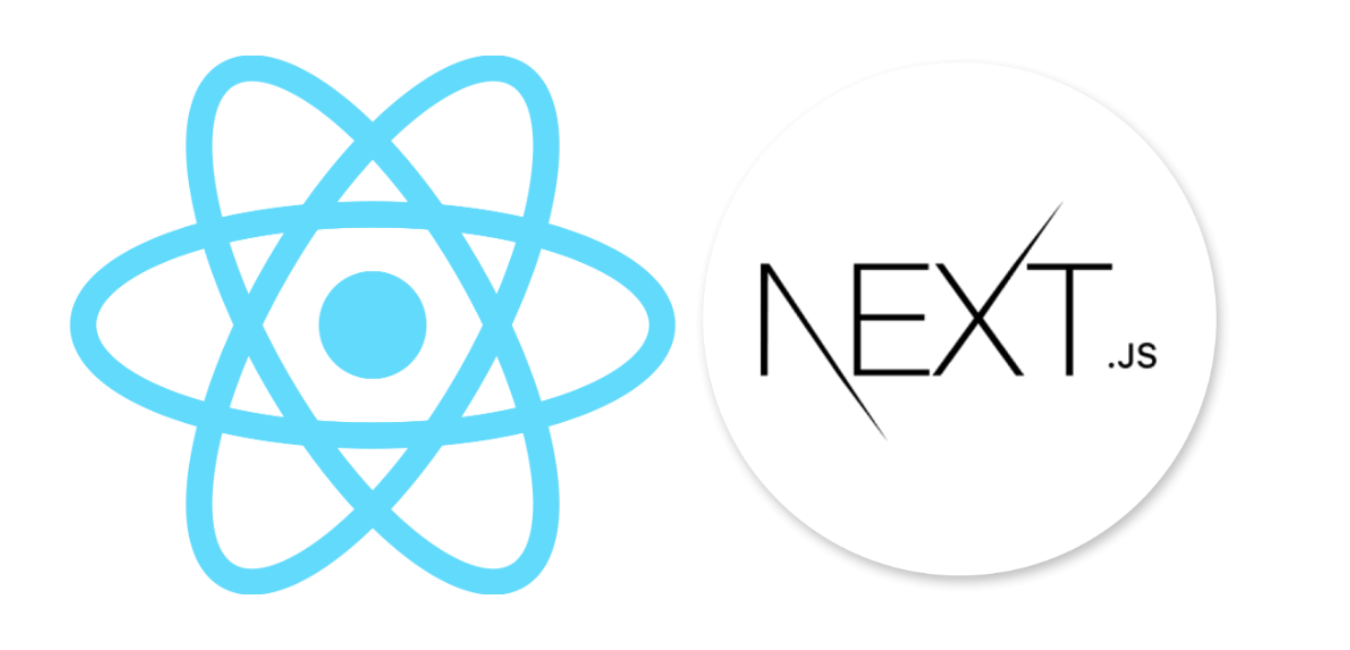
Tailwind With Nexjs
Tailwind CSS is a versatile, low-level CSS framework that offers a wide array of customizable building blocks for creating unique designs without the hassle of overriding pre-defined styles. Integrating Tailwind CSS with Next.js can significantly streamline your development workflow, making it easier to build responsive and visually appealing user interfaces. Let’s dive into how you can set up Tailwind CSS in your Next.js project.
Prerequisites
Before we dive into the setup, ensure you have the following prerequisites:
- Node.js: Installed on your machine.
- Basic knowledge of JavaScript and React: Familiarity with these technologies will be beneficial.
- Familiarity with Next.js: Understanding its core concepts will help you get the most out of this integration.
Table of content
- Step 1: Create a Next.js Project
- Step 2: Install Tailwind CSS Using the CLI
- Step 3: Configure tailwind.config.js
- Step 4: Create Your Styles
- Step 5: Import Tailwind CSS Styles
- Step 6: Start Your Next.js Project
- Example: Building a Card Component
- Conclusion
Step 1: Create a Next.js Project
If you haven't already, create a new Next.js project using the following command in your terminal:
npx create-next-app my-nextjs-project
Replace my-nextjs-project
with your desired project name. This command sets up a new Next.js project with all the necessary files and configurations.
Step 2: Install Tailwind CSS Using the CLI
Navigate to your newly created project directory using the following command:
cd my-nextjs-project
Next, use the Tailwind CSS CLI tool to install Tailwind CSS and set up the required configuration files using either npm or yarn: Using Npm:
npm install tailwindcss @tailwindcss/postcss postcss
or Using Yarn
yarn add -D tailwindcss postcss autoprefixer
After installation, initialize the Tailwind configuration files:
npx tailwindcss init -p
This command initializes a tailwind.config.js
file and a postcss.config.js
file, which are essential for integrating Tailwind CSS into your project.
Step 3: Configure tailwind.config.js
Open the tailwind.config.js
file in your code editor. Here, you can customize the configuration based on your project requirements. For instance, you can define custom colors, fonts, and other styling options. Below is an example configuration:
module.exports = { content: [ "./pages/**/*.{js,ts,jsx,tsx}", "./components/**/*.{js,ts,jsx,tsx}", // Add more paths here if you have them ], // Other configurations...}
Make sure to include all relevant paths in the content array to ensure Tailwind CSS applies styles correctly.
Step 4: Create Your Styles
Create a new CSS file, such as styles/globals.css
, and include the following Tailwind CSS imports:
/* styles/tailwind.css */@import 'tailwindcss/base';@import 'tailwindcss/components';@import 'tailwindcss/utilities';
These imports ensure that all Tailwind CSS base styles, components, and utility classes are available throughout your project.
Step 5: Import Tailwind CSS Styles
To apply the Tailwind CSS styles globally across your application, import the globals.css
stylesheet in your _app.js
file:
// pages/_app.jsimport '../styles/tailwind.css';
function MyApp({ Component, pageProps }) { return <Component {...pageProps} />;}
export default MyApp;
This step ensures that the Tailwind CSS styles are applied consistently to every route in your application.
Step 6: Start Your Next.js Project
Now that everything is set up, start your Next.js development server using the following command:
npm run dev
Visit http://localhost:3000
in your browser. You should now see your Next.js project with Tailwind CSS styles applied seamlessly.
Example: Building a Card Component
Let's create a simple card component using Tailwind CSS to demonstrate how easy it is to style components with utility-first classes.
Create a Card Component
In your components directory, create a new file named Card.js and add the following code:
// components/Card.jsexport default function Card({ title, description }) { return ( <div className="max-w-sm rounded overflow-hidden shadow-lg bg-indigo-700 p-6"> <div className="px-6 py-4"> <div className="font-bold text-xl mb-2">{title}</div> <p className="text-gray-700 text-base"> {description} </p> </div> <div className="px-6 pt-4 pb-2"> <span className="inline-block bg-indigo-500 rounded-full px-3 py-1 text-sm font-semibold text-gray-200 mr-2 mb-2 hover:bg-indigo-600">#nextjs</span> <span className="inline-block bg-indigo-500 rounded-full px-3 py-1 text-sm font-semibold text-gray-200 mr-2 mb-2 hover:bg-indigo-600">#tailwindcss</span> </div> </div> );}
Use the Card Component
Now, let's use this card component in one of your pages, say index.js:
// pages/index.jsimport Card from '../components/Card';
export default function Home() { return ( <div className="container mx-auto mt-8 bg-indigo-300"> <h1 className="text-3xl font-bold mb-4">Welcome to My Next.js App with Tailwind CSS</h1> <Card title="Tailwind CSS Integration" description="Learn how to integrate Tailwind CSS with Next.js to build beautiful, responsive UIs." /> </div> );}
Preview
Welcome to My Next.js App with Tailwind CSS
Learn how to integrate Tailwind CSS with Next.js to build beautiful, responsive UIs.
Conclusion
Congratulations! You’ve successfully integrated Tailwind CSS into your Next.js project using either the CLI method or manual installation via npm/yarn. By leveraging the power of Tailwind’s utility classes and Next.js features, you can prototype, design, and build responsive user interfaces efficiently.
The card component example demonstrates how easy it is to create styled components using Tailwind CSS. Remember to explore Tailwind CSS’s extensive documentation to discover additional customization options and advanced techniques. With this setup, you’re well-equipped to create visually stunning and highly functional web applications.
Resources
Windframe is a drag and drop builder for rapidly building tailwind css websites and UIs
Start building stunning tailwind UIs!
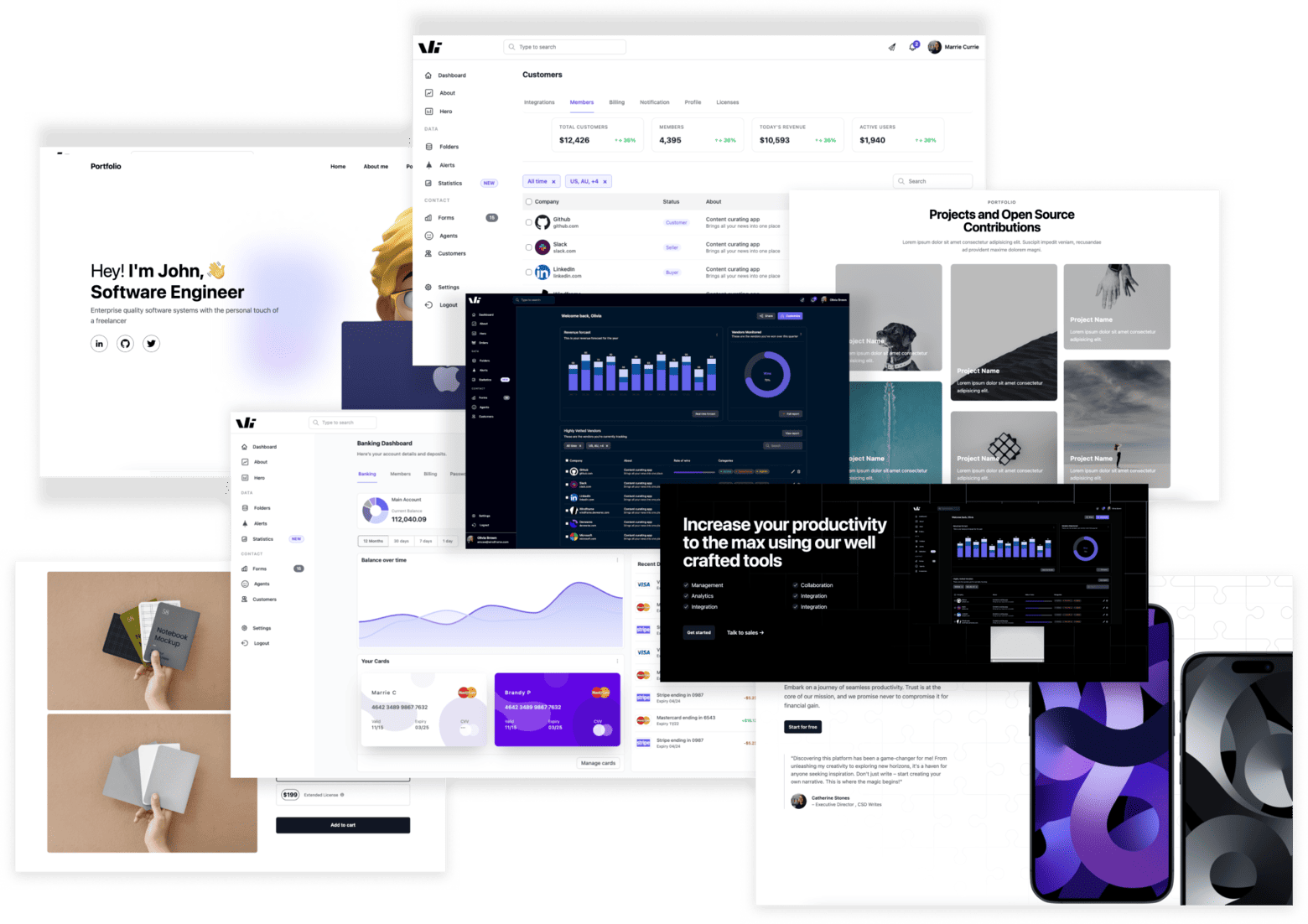