How to install Tailwind CSS with Laravel
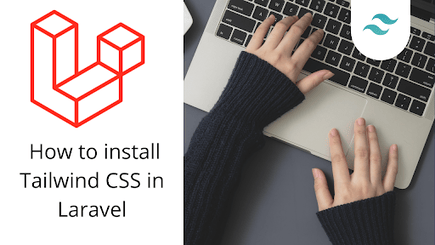
Tailwind in Laravel
If you're a web developer, you probably know how important it is to have a responsive and well-designed website. One tool that can help you achieve this is Tailwind CSS. In this article, we will discuss how to install Tailwind CSS with Laravel, one of the most popular PHP frameworks.
Table of content
- Introduction
- Advantages of using Tailwind CSS with Laravel
- Prerequisites
- Installing Tailwind CSS with Laravel
- Configuring Tailwind CSS
- Include tailwind in your CSS
- Creating a sample application
- Adding custom styles
- Compiling Tailwind CSS
- Conclusion
Introduction
Tailwind CSS is a utility-first CSS framework that provides pre-defined classes to help you design your website. Laravel is a PHP web application framework that provides an elegant syntax and tools to help you build web applications quickly. By combining Tailwind CSS with Laravel, you can create a responsive and visually appealing website in a short amount of time.
Advantages of using Tailwind CSS with Laravel
Here are some advantages of using Tailwind CSS in Laravel:
Tailwind CSS is easy to learn and provides a lot of pre-defined classes that can help you design your website quickly. Laravel's Blade templating engine makes it easy to use Tailwind CSS in your views. Tailwind CSS is highly customizable and provides a lot of options to help you design your website according to your needs. Laravel provides a lot of tools to help you build web applications quickly, and using Tailwind CSS can help you create a responsive and visually appealing website in a short amount of time.
Prerequisites
Before you can install Tailwind CSS with Laravel, you need to have the following:
- Laravel installed on your machine
- Node.js installed on your machine
- NPM installed on your machine
Installing Tailwind CSS with Laravel
To install Tailwind CSS with Laravel, follow these steps:
- Open a command prompt and navigate to your Laravel project directory.
- Run the following command to install Tailwind CSS
npm install tailwindcss
Run the following command to install the Laravel Mix plugin for Tailwind CSS:
npm install laravel-mix-tailwind
Configuring Tailwind CSS
After installing Tailwind CSS, you need to configure it for your Laravel project. To do this, follow these steps:
Create a new file named tailwind.config.js
in your project root directory.
Add the following code to the file:
module.exports = { purge: [], darkMode: false, // or 'media' or 'class' theme: { extend: {}, }, variants: { extend: {}, }, plugins: [],};
Add the following code to your webpack.mix.js
file:
const mix = require("laravel-mix");
require("laravel-mix-tailwind");
mix .js("resources/js/app.js", "public/js") .sass("resources/sass/app.scss", "public/css") .tailwind();
Include tailwind in your CSS
You will need to import the tailwind from the app.css using the path ./resources/css/app.css
. As this was generated by default and replace the original file contents with @tailwind
directives to include Tailwind’s base
, component
, and utilities
styles.
/* ./resources/css/app.css */@import "~tailwindcss/base.css";@import "~tailwindcss/components.css";@import "~tailwindcss/utilities.css";
Creating a sample application
We are going to build a simple
- Create a new Laravel project using the following command:
laravel new sample-project
- Navigate to the project directory and run the following command to start the development server:
php artisan serve
-
Open your web browser and navigate to http://localhost:8000. You should see the default Laravel welcome page.
-
Create a new file named
welcome.blade.php
in the resources/views directory. -
Add the following code to the file:
<body> <div class="bg-gradient-to-br from-purple-900 to-indigo-900 flex flex-wrap items-center justify-center min-h-screen"> <form class="w-full max-w-md bg-white shadow-md rounded px-8 pt-8"> <div class="mb-4"> <label class="text-gray-800 text-sm block font-bold mb-2 pb-2">Username</label> <input class="shadow appearance-none border rounded w-full py-2 px-3 text-gray-700 leading-tight focus:outline-none focus:shadow-outline" id="username" type="text" placeholder="Username"> </div> <div class="mb-6"> <label class="block text-gray-700 text-sm font-bold mb-2">Password</label> <input class="shadow appearance-none border border-red-500 rounded w-full py-2 px-3 text-gray-700 mb-3 leading-tight focus:outline-none focus:shadow-outline" id="password" type="password" placeholder="**************"> <p class="text-purple-500 text-xs italic">Please choose a Password.</p> </div> <div class=" flex items-center justify-between"> <button class="bg-purple-500 hover:bg-indigo-700 text-white font-bold py-2 px-4 rounded focus:outline-none focus:shadow-outline" type="button"> Sign in </button> <a class="inline-block align-baseline font-bold text-sm text-indigo-500 hover:text-indigo-900" href="#"> Forgot Password? </a> </div> <div class="px-8 pt-8"> <p class="text-center text-gray-900 text-xs font-bold">@2023 Devwares develop. All rights reserved. </p> </div> </form>
</div> </body></html>
Below is how our webpage should look once we have saved our file. And reloaded our server.
.
Adding custom styles
Tailwind CSS provides a lot of pre-defined classes, but you may need to add custom styles to your website. To add custom styles, you can create a new file named app.scss
in the resources/sass directory and add your styles to the file.
For example, to add a custom color to your website, you can use the following code:
copycode\$custom-color: #f00;
.btn { background-color: \$custom-color;}
After adding your custom styles, you need to compile the Sass code to CSS code using the following command:
npm run dev
Compiling Tailwind CSS
After making changes to your Tailwind CSS configuration or custom styles, you need to compile the CSS code using the following command:
npm run dev
This will compile your Sass code to CSS code and generate a new app.css
file in the public/css directory.
Conclusion
Tailwind CSS is a powerful CSS framework that can help you create a responsive and visually appealing website quickly. By combining Tailwind CSS with Laravel, you can take advantage of Laravel's tools and utilities to build web applications quickly and efficiently.
In this article, we discussed how to install Tailwind CSS with Laravel, how to configure Tailwind CSS, and how to use Tailwind CSS with Laravel's Blade templating engine. We also discussed how to add custom styles and how to compile Tailwind CSS.
If you're a web developer, we highly recommend using Tailwind CSS with Laravel to create professional-looking websites quickly and efficiently.
Windframe is a drag and drop builder for rapidly building tailwind css websites and UIs
Start building stunning tailwind UIs!
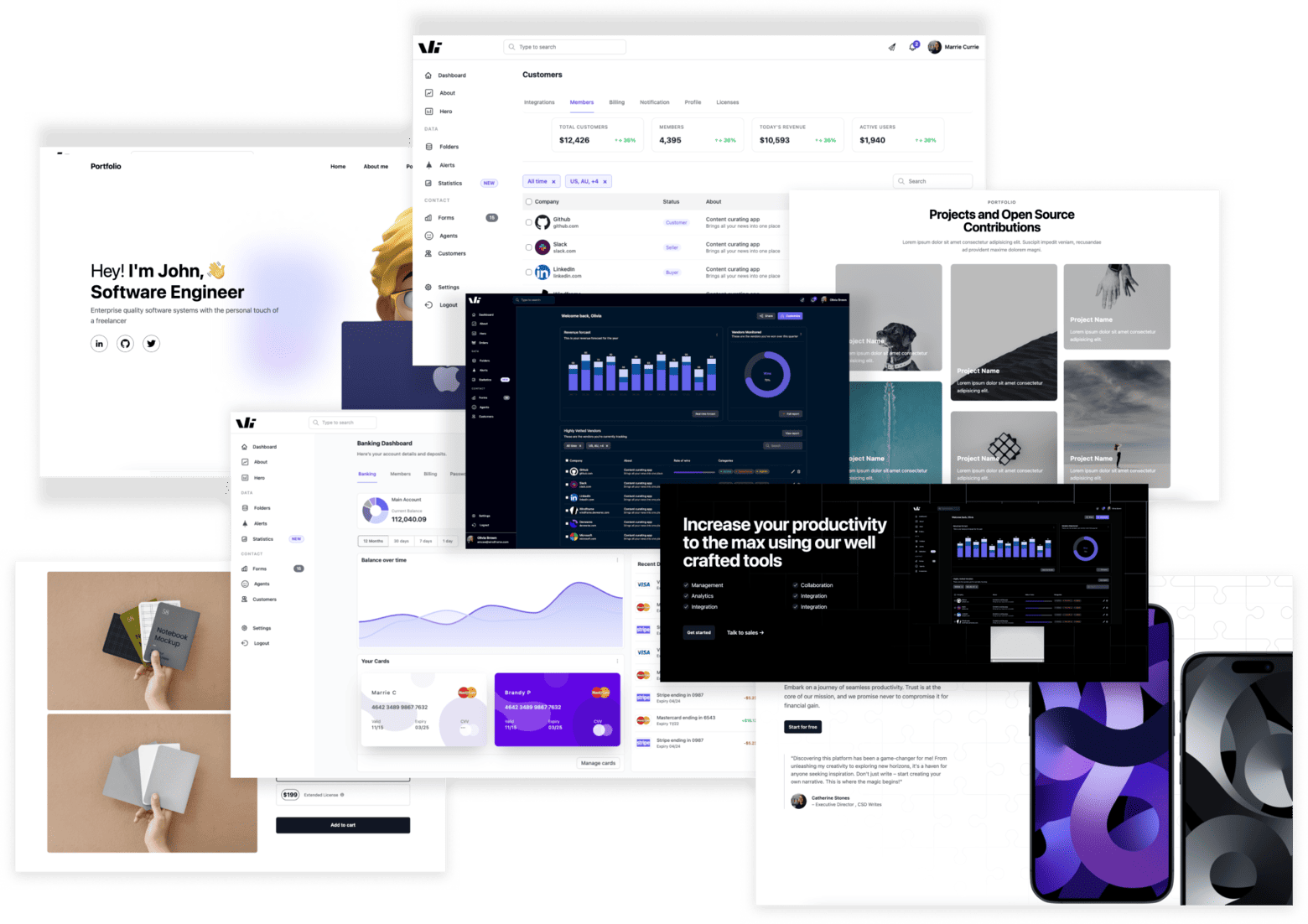